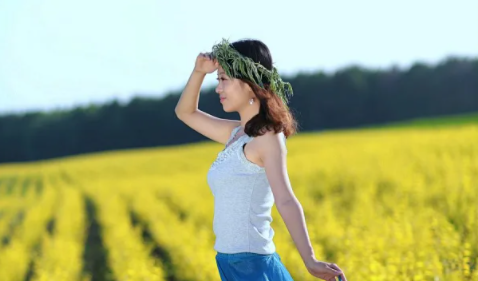
# 人狗大战Java代码实现
在这篇文章中,我们将通过一个简单的Java程序来模拟一个“人狗大战”的小游戏。游戏中,人和狗分别有自己的血量和攻击力,玩家可以选择攻击或防御。
代码实现
java
import java.util.Random;
import java.util.Scanner;
class Creature {
String name;
int health;
int attackPower;
Creature(String name, int health, int attackPower) {
this.name = name;
this.health = health;
this.attackPower = attackPower;
}
void attack(Creature target) {
target.health -= this.attackPower;
System.out.println(this.name + " attacked " + target.name + " for " + this.attackPower + " damage!");
}
boolean isAlive() {
return this.health > 0;
}
void displayStatus() {
System.out.println(this.name + " - Health: " + this.health);
}
}
public class DogWarGame {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
Creature player = new Creature("Player", 100, 20);
Creature dog = new Creature("Dog", 80, 15);
while (player.isAlive() && dog.isAlive()) {
player.displayStatus();
dog.displayStatus();
System.out.print("Choose action (1: Attack, 2: Defend): ");
int choice = scanner.nextInt();
if (choice == 1) {
player.attack(dog);
} else if (choice == 2) {
System.out.println(player.name + " is defending!");
continue;
}
if (dog.isAlive()) {
dog.attack(player);
}
}
if (player.isAlive()) {
System.out.println("You win!");
} else {
System.out.println("You are defeated!");
}
scanner.close();
}
}
代码解析
在这个程序中,我们定义了一个名为`Creature`的类,该类有属性:名字、生命值和攻击力。`attack`方法用于实现攻击逻辑,而`isAlive`方法用来判断生物是否存活。主类`DogWarGame`中,我们创建了一个玩家和一只狗,并使用控制台输入实现了战斗逻辑。
游戏以轮换的方式进行,玩家可以选择攻击或防守。当一方的生命值降至0时,游戏结束。这是一个基础的示例,可以扩展更多功能,例如增加道具、技能等,提升游戏的趣味性和复杂度。通过这个简单的程序,我们展示了Java编程的基础以及面向对象的思想。